Create a secure web server in node.js
Introduction
My website is built using node.js and express. The content management system is using a 'headless' CMS called Contentful.
I wanted my website to not only work on HTTPS, but also redirect users who come in using unencrypted HTTP to the appropriate HTTPS version of the site. The below example shows how this is done in node.js.
Sample Code
Here's all of the code required in order to make this web server work using node.js. For this project you will need to create a self-signed SSL cert, or use a cert that you already own.
// dependencies
var express = require('express');
var http = require('http');
var https = require('https');
var fs = require("fs");
var app = express();
/* GET home page. */
app.get('/', function (req, res) {
res.send("HELLO WORLD");
});
// file location of private key
var privateKey = fs.readFileSync( 'private.key' );
// file location of SSL cert
var certificate = fs.readFileSync( 'ssl.crt' );
// set up a config object
var server_config = {
key : privateKey,
cert: certificate
};
// create the HTTPS server on port 443
var https_server = https.createServer(server_config, app).listen(443, function(err){
console.log("Node.js Express HTTPS Server Listening on Port 443");
});
// create an HTTP server on port 80 and redirect to HTTPS
var http_server = http.createServer(function(req,res){
// 301 redirect (reclassifies google listings)
res.writeHead(301, { "Location": "https://" + req.headers['host'] + req.url });
res.end();
}).listen(80, function(err){
console.log("Node.js Express HTTPS Server Listening on Port 80");
});
Github Repository
It took me awhile to figure this out so I wanted to share the code so anyone can find it. I created a basic node.js web application and put the code on GitHub for anyone to use.
GIT REPO: expresswithSSL
Using the SSL Cert
In order to make this work, you must first obtain an SSL Certificate for your domain. If you need an SSL cert for your website, we sell them! Our IT Services Site - SSL Certs or at any other reputable provider of SSL certificates. Or you could also generate your own self-signed cert.
You will have to generate an encryption key called a CSR, or Certificate Signing Request at the time you create your SSL certificate. The private key that comes with the cert will be saved in your file system. In the example source code, this file will be called "private.key", and is referenced from the node.js code.
Also, the downloaded SSL certificate will be required. I saved that file as SSL.crt in the file system in the example code.
Install and Run the App
From there, you should be able to perform a git pull of this code to your machine, and install the node.js app as follows.
npm install
After that, the web server can be started using the following command.
node app.js
You should see a message that the server is running on both ports 443 and 80. The reason the web app is running on two ports is because port 443 is HTTPS and port 80 is HTTP. This ensures that visitors will arrive on your site even if they don't explicitly type "https://" at the beginning of the URL.
Redirect from HTTP to HTTP with Express
Port 80 will redirect all traffic to the HTTPS version of the website. The below example shows how this is done in node.js.
In order to test this, you will need to navigate to http://localhost or https://localhost in your browser and make sure that the web page says "HELLO WORLD".
It's important to note that unless you are serving up the application from the domain that is registered using the cert, even using HTTPS will show an "insecure" message in Chrome. For example, see how we're getting the error message using "localhost" as the URL.
You will need to install this on a public facing server and set up DNS for your domain name to map to your server's address. Only then will the "Not Secure" message be removed.
Conclusion
I hope that you found this article helpful! I know that when I put it together it was hard to find a good example of how to create an HTTPS server with Node.js and also redirect HTTP to HTTP using Express.
Thank you for reading!
If you are interested in another article by us, check out this one! Building a OAuth Server with Node.js
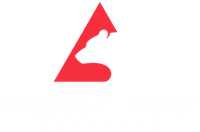
Recent Articles
We create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!